This is a very common operation performed in data management. When it comes to deletion of files it is always recommended to have file check logic in place before you attempt to delete files.
You can delete files using the FDELETE() function. But before that you must verify if a file exists or not using the FILEEXIST() function.
FILEEXIST Function:
FILEEXIST returns 1 if the external file exists and 0 if the external file does not exist. The specification of the physical name for filename varies according to the operating environment. FILEEXIST can also verify a directory’s existence.
FILEEXIST(file-name)
FDELETE Function:
The FDELETE function allows you to delete directories or files directly controlled by SAS. Alternatively you can submit the appropriate operating system command to delete the directory/file.
FDELETE(fileref | directory)
You can assign filerefs by using the FILENAME statement, the FILENAME external file access function, or the FILENAME statement, FTP, Catalog, Hadoop, WebDAV, and ZIP access methods.
The following examples are demonstrated using SAS Studio.
Did you know? How To Get SAS Studio Access For Free?
Example 1: How To Check Files If It Exists Using SAS Code
The following SAS macro checks for given file existence and prints the message in the log. FILEEXIST Function is used to check for sample file qr_code.png.
The qr_code.png file is already present in the directory hence the expected result is to print the relevant message.
/* check if files exists */
%macro checkFiles(file);
%if %sysfunc(fileexist(&file)) ge 1 %then
%do;
%put "The file &file exists";
%end;
%else
%put "The file &file does not exist";
%mend checkFiles;
/* call the macro with file name */
%let file= "/home/u61950255/Files_Out/qr_code.png";
%checkFiles(&file);
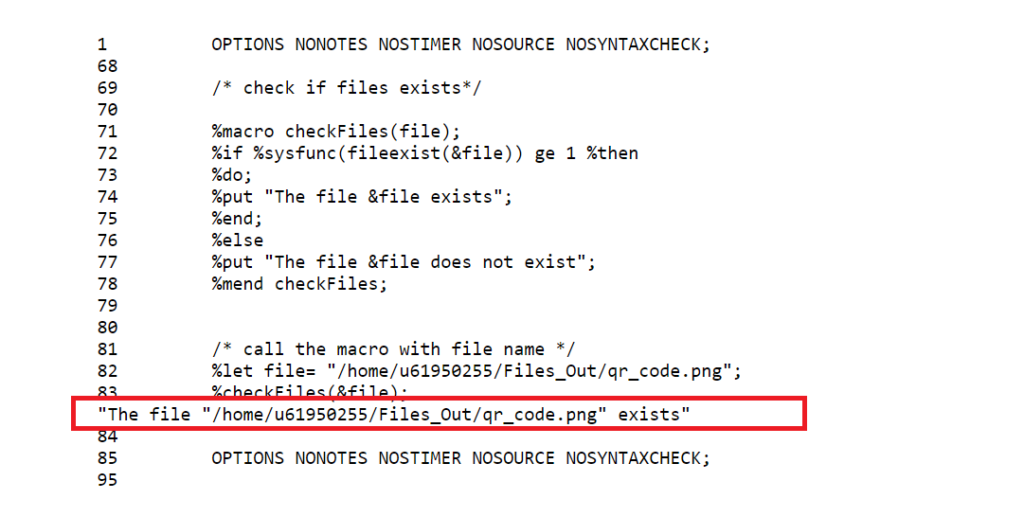
Example 2: How To Check and Delete Files If It Exists Using SAS Code
The following SAS macro checks and deletes the given file and prints the relevant message in the log. FILEEXIST Function is used to check for sample file qr_code.png existence and deletes it using FDELETE function.
The qr_code.png file is already present in the directory hence the expected result is to print the relevant message and delete the file.
/* check and delete files if it exists using sas code */
%macro deleteFiles(file);
%if %sysfunc(fileexist(&file)) ge 1 %then
%do;
%put "The file &file exists, deleting it...";
%let rc=%sysfunc(filename(temp, &file));/* define file reference (i.e. temp)*/
%let rc=%sysfunc(fdelete(&temp)); /* delete file using file reference */
%end;
%else
%do;
%put "The file &file does not exist";
%put "Nothing to do!";
%end;
%mend deleteFiles;
/* call the macro with file name */
%let file= "/home/u61950255/Files_Out/qr_code.png";
%deleteFiles(&file);
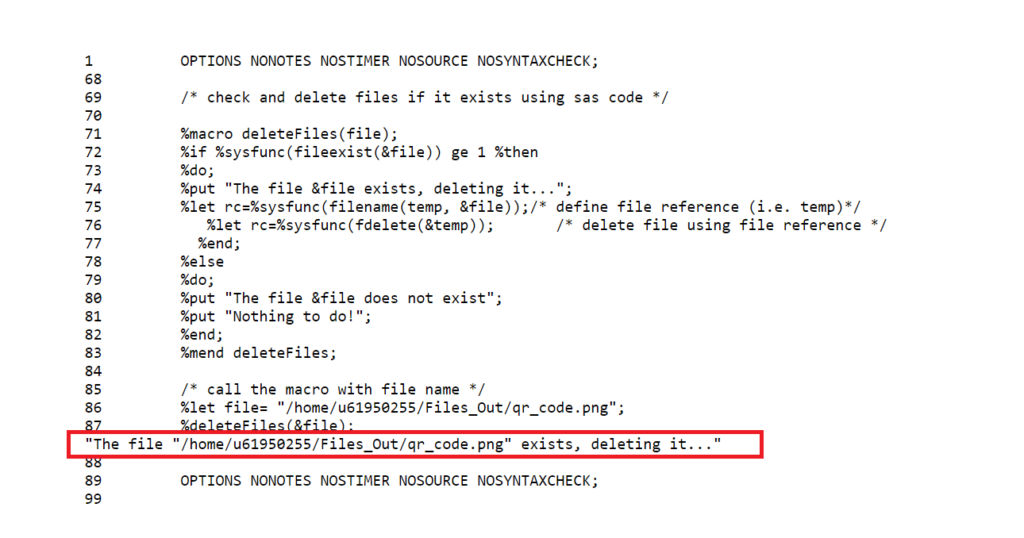
Did you know? How To Create QR Code using SAS Code.
FAQ
You can use the FDELETE function to delete an external file using a fileref. The syntax is FDELETE(fileref), where fileref is a character constant, variable, or expression that specifies the fileref that you assigned to the external file
You can also use the FDELETE function to delete an empty directory using a fileref or a directory name. The syntax is FDELETE(fileref | directory), where fileref is a character constant, variable, or expression that specifies the fileref that you assigned to the directory
To delete a non-empty directory using SAS code, you can use the FDELETE function to delete each file in the directory, and then delete the directory itself.
You can use the DOPEN, DNUM, and DREAD functions to get the list of files in the directory. You can also use a macro to loop through the files and delete them.