Python offers two primary modes for executing code: Python Interactive Mode and Python Script Mode. Both modes ultimately produce the same results, but they cater to different programming needs and come with their own sets of exceptions and limitations.
Running Python Code
Whether using Interactive Mode or Script Mode, the Python interpreter functions similarly to deliver consistent results. However, each mode has its own nuances and use cases.
If Python is not yet installed on your machine, what are you waiting for? Download it now and get started!
Python Programming Modes:
1. Python Interactive Mode
In Interactive Mode, you can write and execute Python commands directly from the Command Prompt. To start, simply type python and hit Enter. This will launch the Python interpreter, allowing you to enter commands and see immediate results.
Microsoft Windows [Version 10.0.17763.195]
(c) 2018 Microsoft Corporation. Med enerett.
H:>python
Python 3.7.2rc1 (tags/v3.7.2rc1:75a402a217, Dec 11 2018, 23:05:39) [MSC v.1916 64 bit (AMD64)] on win32
Type “help”, “copyright”, “credits” or “license” for more information.
>>>
Type the following print command in your python prompt and press enter to see the result.
The result will appear in next line automatically when you press ENTER.
Here is the print command and its output.

2. Script mode Python Programming
If you are using command prompt to execute python script, then you must invoke the interpreter with file name as parameter. You may write your python code in any editors – notepad, notepad++ but the file extension should be .py to execute the program by interpreter.
Let’s take same example and try to execute it in script mode. Your script name is blog.py and it has the same code,
print(“This is a sample Python Program”)
We assume that you have Python interpreter set in PATH variable and your file present in the same directory where you are currently at. Now, try to run this program as follows –
$ python blog.py
Your program must be present in the current working directory otherwise you will get this error – “python: can’t open file ‘blog.py’: [Errno 2] No such file or directory”
If everything is fine then this script will produce same output,
This is a sample Python Program
A Longer Python Program
Now we’ll try to run program which has more than one-line code. When we have more than one line of code and try to execute in interpreter mode and see the result.
Let’s try to print this star using interpreter mode.
print(" * ")
print(" *** ")
print(" ***** ")
print(" *** ")
print(" * ")
If we try to enter each line one at a time into the IDLE interactive shell, the program’s output will be intermingled with the statements you type.
For example,
>>> print(" * ")
*
>>> print(" *** ")
***
>>> print(" ***** ")
*****
>>> print(" *** ")
***
>>> print(" * ")
*
>>>
In such scenario the best approach is to type all code into editor, save the program in file (star.py) and run the program.
Most of the time we use an editor to enter and run our Python programs. The interactive interpreter is most useful for experimenting with small snippets of Python code
In this example if we run this program star.py in script mode then each print statement “draws” a horizontal slice of star.
$ python star.py
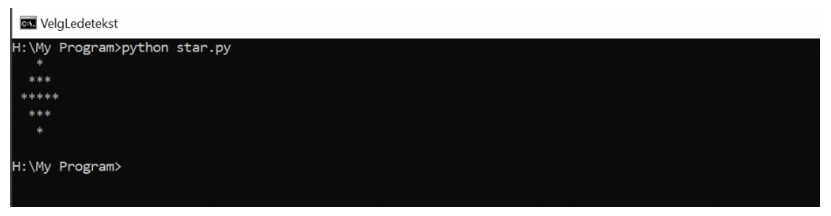
In Python programming, it is more important that no whitespace (spaces or tabs) come before the beginning of each statement.
In Python the indentation of statements is significant and must be done properly. If we try to put a single space before a statement in the interactive shell, we get this ERROR.
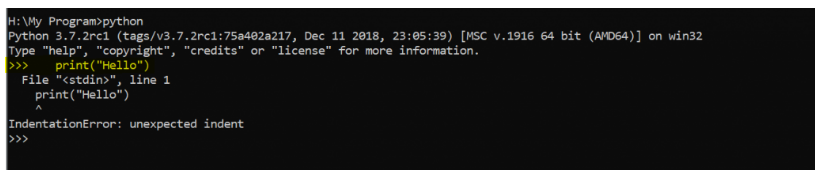
An interpreter reports a similar error when we attempt to run a saved Python program if the code contains such extraneous indentation.
ADDITIONAL RESOURCES:
- How to define and call functions in Python?
- Control statements in Python: break, continue, pass keywords
- If-Else Statement in Python
- For loop and While loop in Python
- Python IDLE, Shell and Command Prompt (Guide)
- Exception handling in Python
- Python Variables, data types, and values
- Python function arguments
- Python interactive mode and script mode programming
- How To Install and Download Python Anaconda?
- Python Raise Keyword
- What is Python? Why it is most loved programming language?
- Write your first Python program!
- Zen of Python and Anti-Zen of Python
- Best Python books to read and learn!