In Python, there are many built-in exceptions that the interpreter raises whenever an unusual situation occurs in the program. While errors and exceptions may seem similar, they function differently.
Python Errors
Errors occur when there is a problem with the code itself, such as syntax issues. When an error happens, the program stops running, and the only way to fix it is by correcting the error in the code. The most common type of error is a syntax error.
For example, the following code contains a syntax error because the colon is missing after the if statement:
var = 100
if var > 10
print("Variable – var =", var)
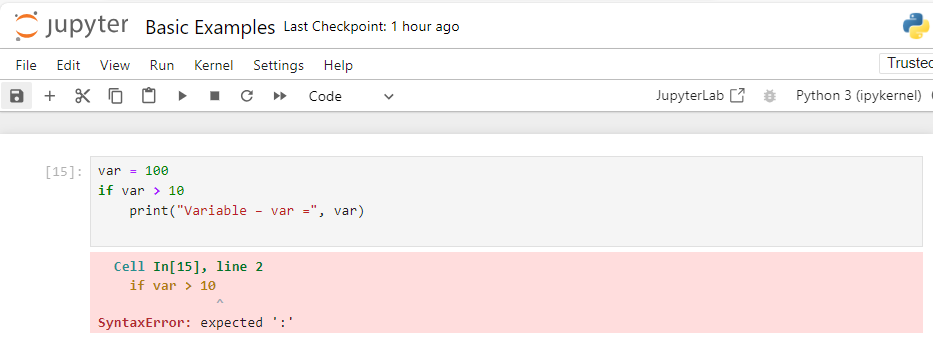
Python Built-in Exceptions
Built-in exceptions are raised by the interpreter or functions when something unexpected happens during code execution. Unlike errors, exceptions can be handled using try/except blocks, allowing the program to continue running after encountering an exception.
For example, trying to divide by zero or accessing a non-existent list index can raise exceptions, but they can be managed within your code.
You can also manually raise exceptions using the raise keyword.
By understanding how to handle both errors and exceptions, you can write more robust and error-resistant Python programs.
Here’s a table that lists some of the most common built-in Python exceptions:
Exception | Description |
---|---|
SyntaxError | Raised when there is an error in Python syntax. |
TypeError | Raised when an operation or function is applied to an object of inappropriate type. |
ValueError | Raised when a function receives an argument of the right type but inappropriate value. |
IndexError | Raised when trying to access an index that is out of range in a list or sequence. |
KeyError | Raised when trying to access a dictionary key that doesn’t exist. |
NameError | Raised when a local or global name is not found. |
ZeroDivisionError | Raised when attempting to divide by zero. |
AttributeError | Raised when an attribute reference or assignment fails. |
ImportError | Raised when an import statement fails to find the module definition or an import fails. |
FileNotFoundError | Raised when trying to open a file that does not exist. |
IOError | Raised when an I/O operation (e.g., file read/write) fails. |
MemoryError | Raised when the program runs out of memory. |
OverflowError | Raised when the result of an arithmetic operation is too large to be expressed within the range. |
RuntimeError | Raised when an error is detected that doesn’t fall into any specific category. |
IndentationError | Raised when there is incorrect indentation in the code. |
UnboundLocalError | Raised when a local variable is referenced before it has been assigned. |
AssertionError | Raised when an assertion statement fails. |
NotImplementedError | Raised when an abstract method that needs to be implemented in a subclass is not implemented. |
These exceptions can be caught and handled in your Python code using try/except blocks to prevent your program from crashing when an unexpected situation occurs.
Summary
In Python, errors occur when there are issues with the code, such as syntax problems, which stop the program from running. Exceptions, on the other hand, are raised during code execution when unexpected situations arise. Unlike errors, exceptions can be handled using try/except blocks, allowing the program to continue running.
Python provides many built-in exceptions like TypeError, ValueError, IndexError, and more, which help in managing and debugging programs effectively. Understanding how to handle these exceptions ensures smoother program execution.
ADDITIONAL RESOURCES:
- How to define and call functions in Python?
- Control statements in Python: break, continue, pass keywords
- If-Else Statement in Python
- For loop and While loop in Python
- Python IDLE, Shell and Command Prompt (Guide)
- Exception handling in Python
- Python Variables, data types, and values
- Python function arguments
- Python interactive mode and script mode programming
- How To Install and Download Python Anaconda?
- Python Raise Keyword
- What is Python? Why it is most loved programming language?
- Write your first Python program!
- Zen of Python and Anti-Zen of Python
- Best Python books to read and learn!