In SAS, dates are stored in numeric and days are calculated by considering the default cut off date which is January 01, 1960 and the date specified. When you’re dealing with SAS dates and calculating time or days, the cut off date is very important.
To calculate age you need two values, the first is date of birth and second one is a current date or a reference date.
1. Calculate number of months
The INTCK function in SAS returns the number of interval boundaries that lie between two SAS dates, times, or timestamp values. Here is the first step where we are
calculating number of months between birthday and current date using following expression:
intck('month',birthday,current_date)
2. Check birthday date and current date
In this step we are deciding whether the current month to be calculated or not. The condition is simple, if the current date is less than the birthday date then don’t count the current month.
The following expression returns either 0 or 1 depending on the dates.
day(current_date) < day(birthday)
3. Divide by 12
Now we have exact months calculated between birthday and current day, let’s just divide by 12 to get the age in the number of years.
The floor function returns the largest integer that is less than or equal to the argument, fuzzed to avoid unexpected floating-point results.
We’re using floor function because even if your age is close to x years it shouldn’t count as X years. It should be X-1.
floor((intck('month',birthday,current_date)-(day(current_date) < day(birthday)))/12)
Calculate Age from Date Of Birth and Current Date
Here is the complete expression to calculate age from date of birth and current date. For demonstration we have hard-coded the birthday date to 11MAY1990.
It can be easily adjusted and replaced with the birthday date variable if you have it in the input data set.
data cal_age;
birthday='11MAY1990'd;
current_date=today();
age=floor((intck('month', birthday, current_date)-(day(current_date) < day(birthday)))/12);
proc print data=cal_age;
run;

Dates are numeric variables and SAS dates are stored in numbers. For better understanding and more clarity, you should use format on date variables.
Let’s print birthday and current_date in readable date formats by adding format statement.
data cal_age;
format birthday current_date date9.;
birthday='11MAY1990'd;
current_date=today();
age=floor((intck('month', birthday, current_date)-(day(current_date) < day(birthday)))/12);
proc print data=cal_age;
run;
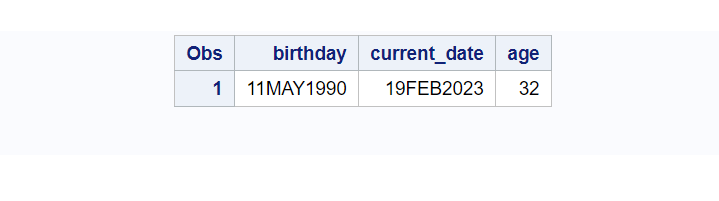
Calculate Age in SAS using Input Data set
Let’s assume you have an input data set with ID, a numeric variable that identifies a person and Birthday variable, where you have the birth date of those individuals.
/* create a new data set */
data birth;
input id birthday date9.;
format birthday date9.;
DATALINES;
1 07DEC2001
2 18FEB1996
3 01JUL1992
4 18FEB1996
5 11SEP1990
6 19MAR1998
7 14JUN1992
8 28AUG1990
9 16MAY1999
10 11APR1994
;
run;
Now add a new variable “AGE” to calculate age for all the rows considering the current date as a base-line. It means you’re calculating their current age.
data cal_age;
set birth;
format current_date date9.;
current_date=today();
age=floor((intck('month', birthday, current_date)-(day(current_date) < day(birthday)))/12);
proc print data=cal_age;
run;

Calculate Age Using SAS Macro
You can place age calculation logic inside the SAS macro and call that macro with birth and date parameters by anytime in your program.
This is very handy when you’re dealing with date calculations on multiple occasions where you don’t want to just calculate the current age but the past or age a few years from now.
Let’s say, you want to calculate what my age was five years back? Or what will be my age 8 years from now?
Here is the tiny SAS macro that can do magic for you:
/* Macro that calculates age */
%macro age(date, birth);
floor((intck('month', &birth, &date) - (day(&date) < day(&birth))) / 12)
%mend;
Let’s say your birth date is 01SEP1990 and you want to calculate your current age using this SAS macro. You just need to call that macro “age” with two parameters: reference date and birth date.
In this case the reference date is today’s date as you want to calculate your current age.
data _null_;
age=%age(date=today(), birth='09SEP1990'd);
put age=;
run;
Output: age=32
Now a simple question to you:
What would be your age on September 01, 2050 ?
It’s easy.
Just replace today() with that specific date. Here it is ‘01SEP2050’
data _null_;
age=%age('01SEP2050'd, '09SEP1990'd);
put 'Your age on September 01, 2050 is: ' age 'years';
run;
Output: Your age on September 01, 2050 is: 59 years
Fun Fact: You can also set back dated date to calculate what was your age on February 15, 2005.
data _null_;
age=%age('15FEB2005'd, '09SEP1990'd);
put 'Your age on February 15, 2005 was: ' age 'years';
run;
Output: Your age on February 15, 2005 was: 14 years
Let’s take the same example where we have a “birth” data set. Instead of directly calculating age in the data step using expression, let’s call SAS macro “%age” to calculate age for you.
/* create a new data set */
data birth;
input id birthday date9.;
format birthday date9.;
DATALINES;
1 07DEC2001
2 18FEB1996
3 01JUL1992
4 18FEB1996
5 11SEP1990
6 19MAR1998
7 14JUN1992
8 28AUG1990
9 16MAY1999
10 11APR1994
;
run;
/* Run this macro */
%macro age(date, birth);
floor((intck('month', &birth, &date) - (day(&date) < day(&birth))) / 12)
%mend;
/* Calculate age using sas macro and insert the age values in a new variable */
data cal_age;
set birth;
format current_date date9.;
current_date=today();
age=%age(current_date, birthday);
proc print data=cal_age;
run;

You can either choose to calculate age directly in the data step or using a sas macro.
The SAS macro is very convenient if you’re calculating on multiple occasions and changing the current base date, otherwise age calculation with simple expression in data step is more than enough.
FAQ
Calculating age in SAS is very easy with the INTCK function. You just need to calculate year difference between date of birth and a reference date using INTCK function.
SAS macro that calculates age by taking two date parameters, date of birth and current date.
/* Macro that calculates age */
%macro age(date, birth);
floor((intck('month', &birth, &date) - (day(&date) < day(&birth))) / 12)
%mend;