You have a SAS data set with few numeric variables and most of them are character variables. Now you want to convert those character variables into numeric variables in the SAS data set.
In this article we have covered how to convert numeric variables into character variables and vice versa. In this article you’ll learn to write SAS macro to identify character variables and convert them into numeric variables.
This is effective where you have 100 odd variables in the SAS dataset and you want to convert char to numeric variables.
The INPUT() and PUT() functions convert values for a variable from character to numeric, and from numeric to character. In SAS a variable can be defined as only one type, so you cannot use the same variable name to convert the values.
RECAP: Convert Character to Numeric in SAS
You can use the INPUT() function in SAS to convert a character variable to a numeric variable.
Syntax:
Numeric_variable = input(char_variable, informat.);
The informat tells SAS how to interpret the data in the original character variable.
Example:
If you have a simple string of digits (numbers only) then you can use informat 8. with input() function.
data new;
char_var = '54321';
numeric_var = input(char_var, 8.);
run;
You can find more examples here.
RECAP: Convert Numeric to Character in SAS
You can use the PUT() function in SAS to convert a numeric variable to a character variable.
Syntax:
character_variable = put(num_variable, format.);
The format tells SAS what format to apply to the value in the original variable. The format must be of the same type as the original variable.
Example:
data new;
num_var = 12345;
char_var = put(num_var,5.);
run;
proc contents data=new; run;
You can find more examples here.
How to Convert ALL character variables into numeric variables in SAS
To solve this example let’s assume you have a data set in which all the variables are character type variables. We will create SAS macro to convert character variables into numeric variables in SAS.
Step 1: Create test SAS data set
/*create a test data set with ALL character variables*/
data chardata;
input x $ y $ a $ b $;
datalines;
41840 0527 1 9
10345 3819 7 4
;
proc contents;
run;
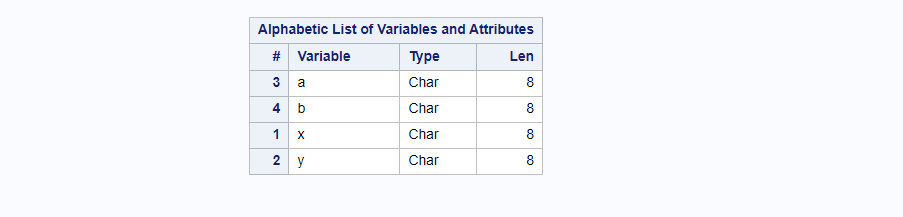
Step 2: Extract Variable names with their position
/*var names and position*/
proc contents noprint data=chardata out=varname_data(keep=name npos);
run;
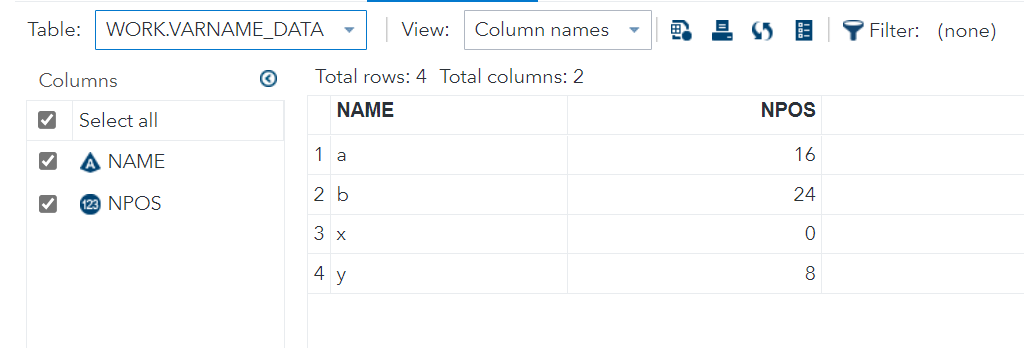
Step 3: Sort data by variable position in order they appear in the data set
/* sort by position */
proc sort data=varname_data;
by npos;
run;
Step 4: Create macro variables: for the name of variables and another set of numeric macro variables as placeholder.
data _null_;
do i=1 to nobs;
set varname_data nobs=nobs end=end;
/*creates macro variables for the name of vars.
in this example: mac1=x, mac2=y, mac3=a, mac4=b */
call symput('mac'||left(put(i, 4.)), name);
/*creates new macro numeric vars as placeholder
num1=num1, num2=num2, num3=num3, num4=num4 */
call symput('num'||left(put(i, 4.)), 'num'||left(put(i, 4.)));
if end then
call symput('end', put(nobs, 8.));
/*determines number of variables, 1 per obs*/
end;
/*assumes none of char vars are larger than 8 bytes*/
run;
Step 5: Convert char to numeric vars: drop and rename variable names so numeric variables can have their original names
/*macro that converts all char vars to numeric, drop and rename vars so numeric vars have original names*/
%macro create;
data numdata(drop= %do i=1 %to &end;&&mac&i %end; /*dropping placeholder temp names*/
rename=(%do i=1 %to &end; &&num&i=&&mac&i %end;)); /* renaming numeric vars to its original names */
%do i=1 %to &end;
set chardata ;
/*convert character to numeric variables and their names resolves to num1-num5*/
&&num&i=input(&&mac&i,5.);
%end;
run;
%mend;
%create;
Step 6: Verify new data set which has numeric variables
/*verify that the new data set contains all numeric vars*/
proc print data=numdata;
run;
proc contents data=numdata;
run;
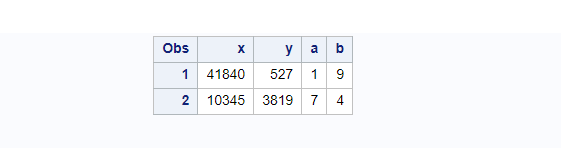
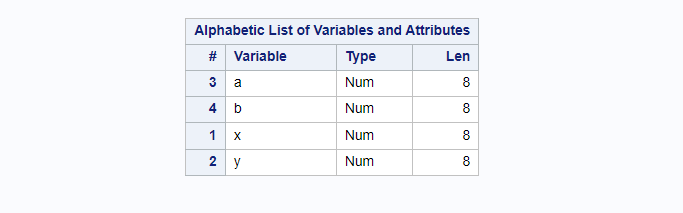
Here is the entire SAS code that converts character variables into numeric variables in SAS data set:
/*create a test data set with ALL character variables*/
data chardata;
input x $ y $ a $ b $;
datalines;
41840 0527 1 9
10345 3819 7 4
;
proc contents;
run;
/*var names and position*/
proc contents noprint data=chardata out=varname_data(keep=name npos);
run;
/* sort by position */
proc sort data=varname_data;
by npos;
run;
/* Create macro variables */
data _null_;
do i=1 to nobs;
set varname_data nobs=nobs end=end;
/*creates macro variables for the name of vars.
in this example: mac1=x, mac2=y, mac3=a, mac4=b */
call symput('mac'||left(put(i, 4.)), name);
/*creates new macro numeric vars as placeholder
num1=num1, num2=num2, num3=num3, num4=num4 */
call symput('num'||left(put(i, 4.)), 'num'||left(put(i, 4.)));
put _ALL_;
if end then
call symput('end', put(nobs, 8.));
/*determines number of variables, 1 per obs*/
end;
/*assumes none of char vars are larger than 8 bytes*/
run;
/*macro that converts all char vars to numeric, drop and rename vars so numeric vars have original names*/
%macro create;
data numdata(drop= %do i=1 %to &end;&&mac&i %end; /*dropping placeholder temp names*/
rename=(%do i=1 %to &end; &&num&i=&&mac&i %end;)); /* renaming numeric vars to its original names */
%do i=1 %to &end;
set chardata ;
/*convert character to numeric variables and their names resolves to num1-num5*/
&&num&i=input(&&mac&i,5.);
%end;
run;
%mend;
%create;
/*verify that the new data set contains all numeric vars*/
proc print data=numdata;
run;
proc contents data=numdata;
run;
FAQ
It is possible to identify character variables in SAS data set and convert them into numeric variables.
Steps to follow:
- Step 1: Create test SAS data set
- Step 2: Extract Variable names with their position
- Step 3: Sort data by variable position in order they appear in the data set
- Step 4: Create macro variables: for the name of variables and another set of numeric macro variables as placeholder.
Step 5: Drop and rename variable names so numeric variables can have their original names
- Step 6: Verify new data set which has numeric variables
Using “proc contents” SAS procedure it is possible to list the character or numeric variables present on any SAS data set.
Extract char variable names with their position
/*list char variable names and position*/
proc contents noprint data=chardata out=varname_data(keep=name npos);
run;