In SAS you can easily extract characters from a string using SUBSTR() or SUBSTRN() functions. But it only works with the character variable.
To extract last 4 digits or any number of digits from a numeric variable, you need to convert the input from numeric variable to character variable in order to use substr function.
You have to do this conversion but it is very straight forward. Extracting last N digits from numeric variable as easy as extracting last N characters from a string.
Extract Last N Digits From A Numeric Variable
For the demonstration purpose we have created employee data set with one numeric variable employeeID.
Create employee data set:
data employee; input employeeID; datalines; 1035423413 1154294133 1205429432 1835413551 2640313091 ; run;
From the above data set you want to extract last 4 digits (highlighted) from a numeric variable employeeID. In order to use substr() function you need to first convert numeric variable into character variable using PUT() function.
PUT(variable, informat);
After extracting last 4 digits you can put it into a separate numeric variable hence you need to convert extracted last 4 digits into numeric variable using INPUT() function.
INPUT(variable, format);
Use SUBSTR() Function To Extract Last 4 Characters
Once you have numeric variable converted into character, you can pass it as an input string, specify starting position and number of char to read.
Syntax:
SUBSTR(char variable, starting position, no of char to read);
Back to our previous example, you can use following code to extract last 4 digits from a numeric variable employeeID.
data employee;
input employeeID;
datalines;
1035423413
1154294133
1205429432
1835413551
2640313091
;
run;
data new_employee;
set employee;
employeeID_char = put(employeeID, 10.); /* Convert numeric into char */
last_four_digits = input(substrn(employeeID_char,length(employeeID_char)-3,4),8.);
drop employeeID_char;
run;
proc print data=new_employee;
title 'Example: Extract last 4 digits from a numeric variable in SAS';
run;
Output:
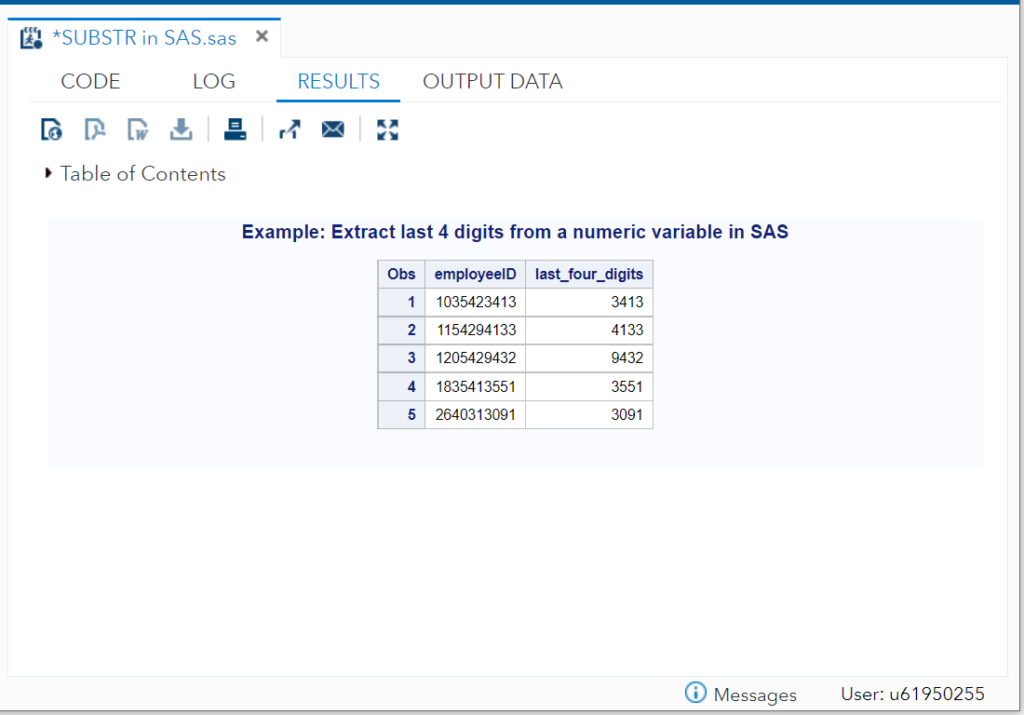
In case if there is any missing values on numeric variable then you might encounter error with this code. You can solve this problem by using SUBSTRN() function instead SUBSTR(). Here is the classic example of this scenario.
FAQ
Yes but you need numeric to character conversion. SUBSTR() function only works with character variable hence you can’t input numeric variable.
First, you need to convert your numeric variable into character variable using PUT() function and then pass it into substr() function to extract last/first N digits.
No. substr() function in SAS only works with character variable. But you can convert numeric variable into char variable using PUT() function before passing it to SUBSTR() function to extract characters from the input string.
This way you can use substr() function with a numeric variable.