In Python, data types, variables, and values are essential elements of any code. They allow you to store, manipulate, and interact with data effectively.
Python Variables
When defining variables in Python, there are some important rules to follow:
- Variable names must be at least one character long.
- The first character must be an alphabetic letter (either uppercase or lowercase) or an underscore (_). For example: AxyBSNDmkerdsladCPFAEVXZkior.
- Remaining characters can include letters, digits, or underscores (e.g., AxyBSNDmkerdsladCPFAEVXZkiorTd_02538923).
- Special characters (like @, %, or –) and spaces are not allowed in variable names.
- Reserved words like class, def, or for cannot be used as variable names since they have special meanings in Python.
- Variable names are case-sensitive. For example, variable and Variable are considered different variables.
Examples of valid variable names: x, xY2, TekkieHead, _Myblog, _2days_BLOG, BLOG.
Examples of invalid variable names:
5
(cannot start with a digit)@_Blog
(special characters not allowed)Tekkie%Head
(special characters not allowed)Python-Program
(dash is not allowed)Class
(reserved word)Good Read
(spaces not allowed)
Examples:
x = 1000
print(x) # Output: 1000
TekkieHead = "Friend of Python"
print(TekkieHead) # Output: 'Friend of Python'
TEKKIEHEAD # Error: 'TEKKIEHEAD' is not defined
Tekkie%Head = "Special character in variable name" # Error: Invalid syntax
Python Keywords as Variable Names
Python has 33 reserved keywords (e.g., print, str, int, type), which are reserved for specific operations and should not be used as variable names. While you technically can reassign these keywords as variables, it is generally discouraged as it can create confusion and errors in your code.
Python Reserves 33 Keywords
and | del | from | None | True |
---|---|---|---|---|
as | elif | global | nonlocal | try |
assert | else | if | not | while |
break | except | import | or | with |
class | false | in | pass | yield |
continue | finally | is | raise | def |
for | lambda | return |
Values and Data Types
Values are the basic data units that your program works with, such as numbers, strings, or more complex objects. Each value in Python has a specific data type, which determines how it can be used and manipulated.
Some common data types in Python include:
- Integer (int): Whole numbers, e.g., 42
- String (str): Sequences of characters, e.g., “Hello”
- Float (float): Numbers with decimal points, e.g., 3.14
To determine the data type of a variable or value, you can use the type() function. For example:
x = 42
print(type(x)) # Output: <class 'int'>
y = "Hello"
print(type(y)) # Output: <class 'str'>

In Python, variables do not need to be declared with a specific type. You can simply assign a value to a variable, and Python will automatically determine its data type.
If you later assign a new value of a different type to the same variable, Python will update the data type accordingly:
x = 42 # x is an integer
x = "Hello" # Now x is a string
This flexibility allows Python to handle variable assignments and reassignments without needing explicit type declarations.
Python take cares of everything when you assign the value. When we try to reassign the value from int to string, python changes the variable data types internally.
In below schematic diagram see how variable assignment and reassignment happens in Python.
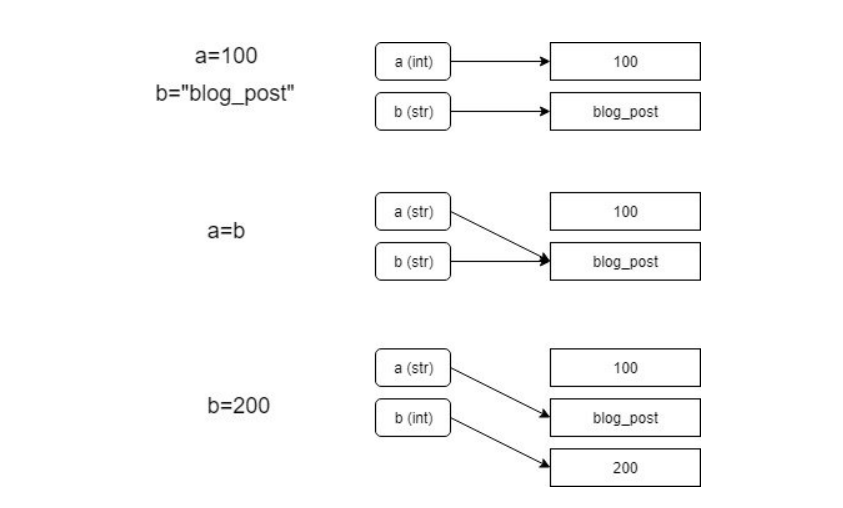
Summary
In Python, variables are named storage locations that follow specific rules for naming, such as starting with a letter or underscore and avoiding special characters or reserved keywords. Python’s data types, like integers, strings, and floats, define the nature of values that variables hold.
Python handles variable types dynamically, allowing flexible reassignment without the need for explicit declarations. Understanding these basics is crucial for writing efficient Python code.
ADDITIONAL RESOURCES:
- How to define and call functions in Python?
- Control statements in Python: break, continue, pass keywords
- If-Else Statement in Python
- For loop and While loop in Python
- Python IDLE, Shell and Command Prompt (Guide)
- Exception handling in Python
- Python Variables, data types, and values
- Python function arguments
- Python interactive mode and script mode programming
- How To Install and Download Python Anaconda?
- Python Raise Keyword
- What is Python? Why it is most loved programming language?
- Write your first Python program!
- Zen of Python and Anti-Zen of Python
- Best Python books to read and learn!