Python Functions
In Python programming, a function is a named block of code designed to perform a specific task. Functions help organize and reuse code efficiently. You define a function in Python using the def keyword. Essentially, a function acts as a reusable package of code that can be called whenever needed in your program.
Python provides many built-in functions that are available by default. Additionally, you can create your own custom functions using the def keyword.
Built-in Functions
Python’s built-in functions are pre-defined in the Python standard library, so you don’t need to import any additional modules to use them.
You’ve encountered several of these functions throughout your Python journey, starting from simple examples like printing “Hello, World” to more complex programs.
For example, the print() function, which you’ve probably used countless times, is a built-in function.
Other commonly used built-in functions include input(), eval(), int(), float(), range(), and type(). These functions are part of the standard library and can be accessed at any time. However, for some built-in functions, you may need to import specific modules at the beginning of your code.
User-defined Functions
User-defined functions are those that you create yourself to perform specific tasks. These are defined using the def keyword.
The concept of functions revolves around two key aspects: defining the function and calling the function with the necessary parameters.
Syntax:
# Define a Python function using the def keyword
def FunctionName(parameters):
# Code block
...
# Call the Python function
FunctionName(parameters)
Let’s look at a simple example of a user-defined function, show(), which displays a predefined message when called:
# Define the show() function
def show():
print("Show function executed")
# Call the show() function
show()
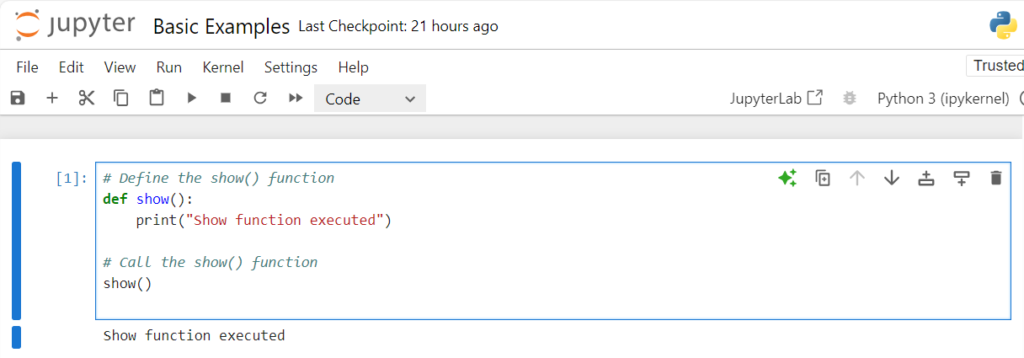
In this example, show() is a user-defined function, but it uses the built-in print() function within it. When you call the show() function, it executes the code inside it. Similarly, when the print() function is called, Python executes the underlying code in the standard library.
Python’s standard library contains many pre-written functions that you can use directly. Whether a function is built-in or user-defined, certain rules need to be followed to ensure the code executes correctly and can be reused efficiently.
Example: Adding Two Numbers Using a Function
Here’s an example of a simple Python function that adds two numbers provided by the user:
# Define the add() function
def add(a, b):
result = a + b
print("Addition of two numbers:", result)
# Take input from the user
a = int(input("Enter First Number: "))
b = int(input("Enter Second Number: "))
# Call the add() function to perform the addition
add(a, b)
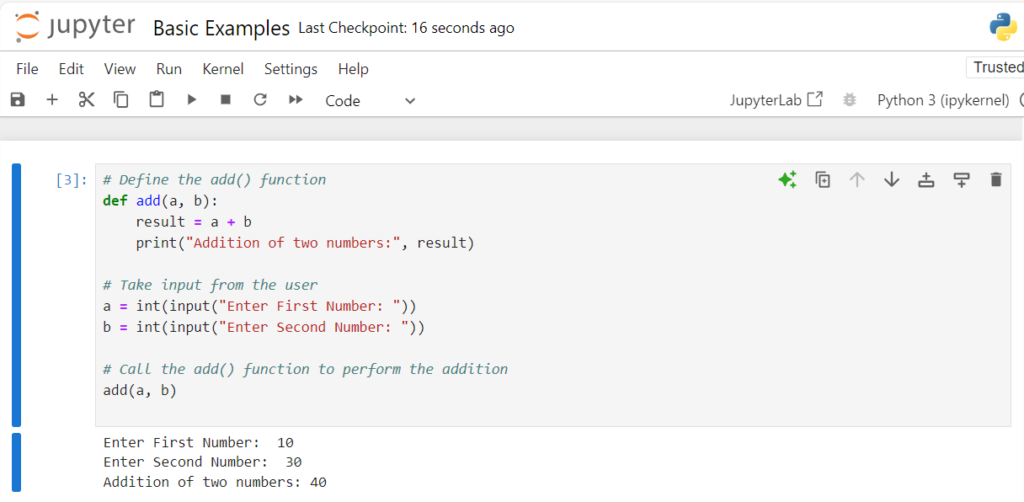
In this example, the add() function takes two numbers as input, adds them, and displays the result. The user provides the input values, and the function performs the addition when called.
Summary
In Python, a function is a named block of code designed to perform a specific task. Functions are defined using the def keyword and can be either built-in (predefined in the Python standard library) or user-defined (created by the programmer).
Built-in functions like print(), input(), and range() are readily available, while user-defined functions allow custom tasks to be performed. Functions help in organizing and reusing code efficiently. The key aspects of functions are defining them and calling them with appropriate parameters.
ADDITIONAL RESOURCES:
- Control statements in Python: break, continue, pass keywords
- If-Else Statement in Python
- For loop and While loop in Python
- Python IDLE, Shell and Command Prompt (Guide)
- Exception handling in Python
- Python Variables, data types, and values
- Python function arguments
- Python interactive mode and script mode programming
- How To Install and Download Python Anaconda?
- Python Raise Keyword
- What is Python? Why it is most loved programming language?
- Write your first Python program!
- Zen of Python and Anti-Zen of Python
- Best Python books to read and learn!