An exception in Python occurs when a runtime error happens, even if the code logic is correct. These situations, known as exceptions, can cause the program to terminate unless properly handled. Python provides a mechanism for handling such errors using the try, except, else, and finally blocks.
- try: Contains code that might raise an exception.
- except: Catches and handles the exception.
- else: Executes when no exception is raised.
- finally: Always runs after the try and except blocks, regardless of whether an exception occurred.
It’s important to understand that a Python exception or error doesn’t always indicate a problem with your logic or algorithm. There are situations where your program is correct, but a run-time error occurs. This is known as an exception.
In the past, these run-time errors would cause a program to terminate. Instead of allowing this, the program can be designed to detect and handle the error intelligently. This process is known as exception handling.
Python provides a standard mechanism for handling run-time errors, known as exception handling. This allows programmers to deal with errors like dividing by zero, converting a non-numeric string to an integer, accessing a list with an invalid index, or referencing an object that is None
.
Here are a few common Python exceptions:
1. ZeroDivisionError: Division by zero
If you have a program that performs calculations, and the user inputs a zero as the divisor, the program will terminate with a ZeroDivisionError.
N1 = int(input("Enter first number (N1): "))
N2 = int(input("Enter second number (N2): "))
Result = N1 / N2
print("Result:", Result)
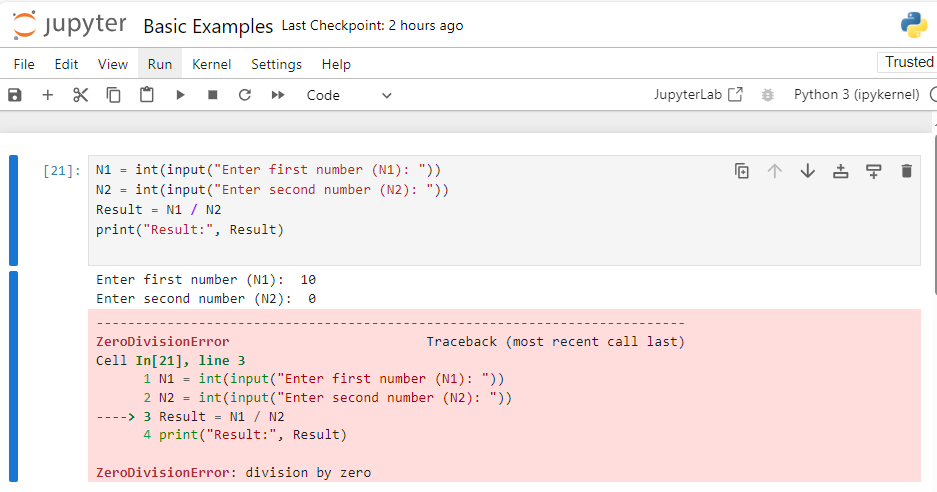
2. ValueError: Invalid literal for int()
If the user inputs a non-numeric value when the program expects an integer, a ValueError will occur. For example, if the user types “five” instead of “5”, the program will throw this exception.
N1 = int(input("Enter first number (N1): "))
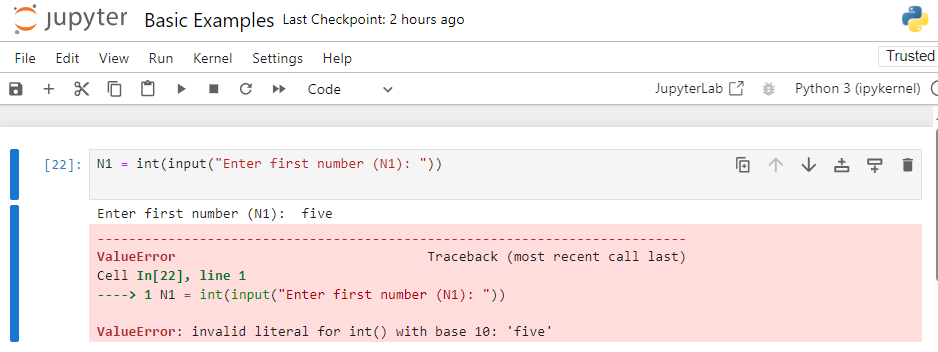
3. OverflowError: Integer division result too large for a float
If the user inputs a number that’s too large to be handled during a calculation, Python will raise an OverflowError. This occurs when the result of an operation is beyond the limits that Python can handle for floating-point numbers.
N1 = int(input("Enter a very large number for N1: "))
N2 = 1
Result = N1 / N2
print("Result:", Result)
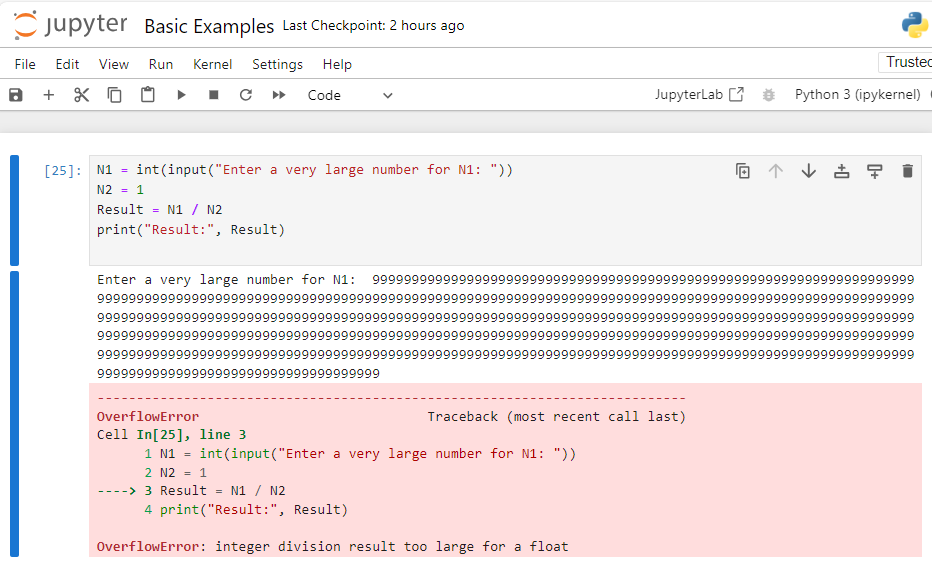
Handling Exceptions in Python
Python offers built-in mechanisms to handle exceptions through the use of the try, except, else, and finally blocks. This allows you to catch potential errors and handle them gracefully instead of letting your program crash.
1. Try and Except
You can use a try block to wrap the code that might raise an exception. If an exception occurs, the except block catches it and provides a way to handle the error.
try:
N1 = int(input("Enter first number (N1): "))
N2 = int(input("Enter second number (N2): "))
Result = N1 / N2
print("Result:", Result)
except ZeroDivisionError:
print("Error: You cannot divide by zero.")
except ValueError:
print("Error: Please enter a valid number.")
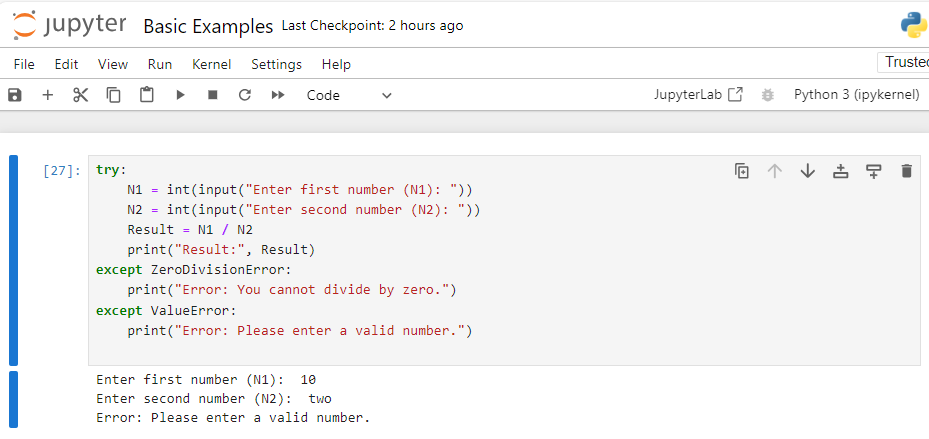
2. Else
The else block executes only if no exceptions were raised in the try block. It’s useful when you want to run some code after a successful try.
try:
N1 = int(input("Enter first number (N1): "))
N2 = int(input("Enter second number (N2): "))
Result = N1 / N2
except ZeroDivisionError:
print("Error: You cannot divide by zero.")
except ValueError:
print("Error: Please enter a valid number.")
else:
print("Division successful! Result:", Result)
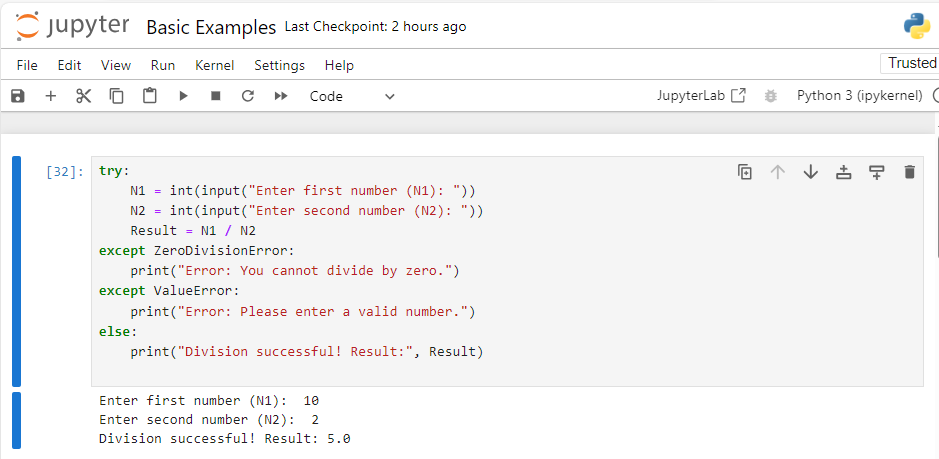
3. Finally
The finally block always executes after the try and except blocks, whether an exception occurred or not. This is useful for cleanup actions that need to occur no matter what.
try:
N1 = int(input("Enter first number (N1): "))
N2 = int(input("Enter second number (N2): "))
Result = N1 / N2
except ZeroDivisionError:
print("Error: You cannot divide by zero.")
except ValueError:
print("Error: Please enter a valid number.")
finally:
print("This block always executes.")
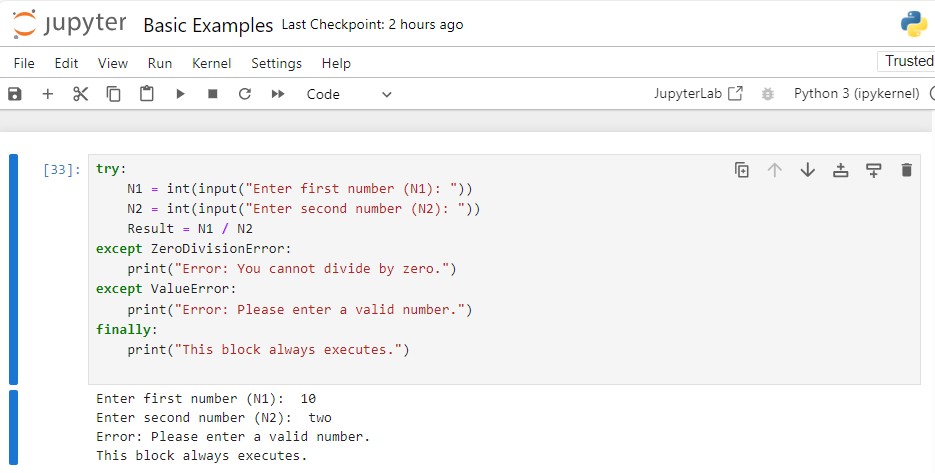
Example: Exception Handling with a Loop
In this example, the user is prompted to input a small integer. If they input an invalid value, the program continues asking until a valid input is received.
x = 0
while x < 100:
try:
x = int(input("Please enter a small integer number: "))
print("x =", x)
except ValueError:
print("Oops! Input cannot be parsed as an integer. Please try again.")
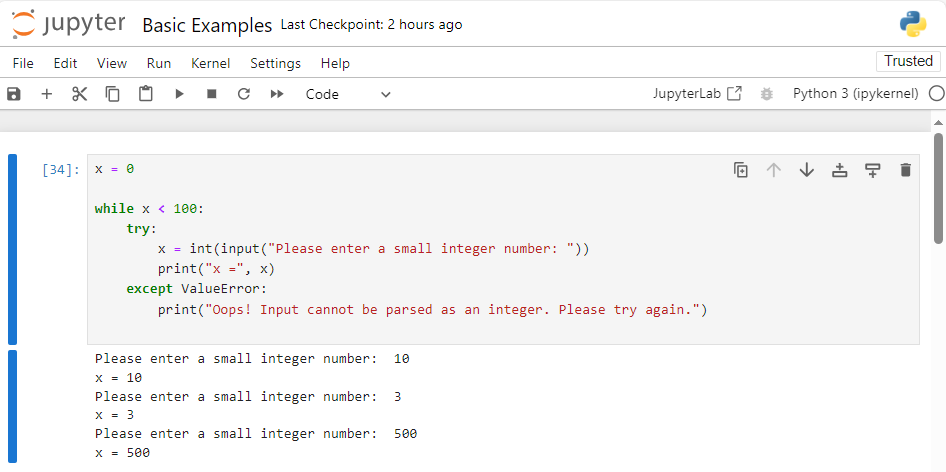
Summary
Using python try, except, else, and finally blocks, you can ensure that your Python programs handle exceptions gracefully without crashing. This makes your code more robust and user-friendly by properly managing errors when they occur.
ADDITIONAL RESOURCES:
- How to define and call functions in Python?
- Control statements in Python: break, continue, pass keywords
- If-Else Statement in Python
- For loop and While loop in Python
- Python IDLE, Shell and Command Prompt (Guide)
- Exception handling in Python
- Python Variables, data types, and values
- Python function arguments
- Python interactive mode and script mode programming
- How To Install and Download Python Anaconda?
- Python Raise Keyword
- What is Python? Why it is most loved programming language?
- Write your first Python program!
- Zen of Python and Anti-Zen of Python
- Best Python books to read and learn!