What Are SAS Arrays?
In SAS, an array is a convenient way of temporarily identifying a group of variables. It’s not a data structure, and the array name is not a variable. An ARRAY statement defines an array. An array reference uses an array element in a program statement.
All variables that are associated with an array must be of the same type, either character or numeric. As a result, arrays are typically referred to as either character arrays or numeric arrays. Variables that are associated with an array do not have to be already existing variables.
If they do not exist within a program data vector (PDV) when the ARRAY statement is compiled, SAS creates them for you.
If I could conclude here is the short definition of SAS Array:
A SAS array is a set of variables of the same type that you want to perform the same operation on. The variables in an array are called elements and can be accessed based on their position (also known as index).
You use the name of the array to reference the set of variables. Arrays are useful to create new variables and carry out repetitive tasks
Syntax of SAS Array
To use arrays in SAS code, first make sure that you understand the basic syntax of the SAS ARRAY statement.
The ARRAY statement consists of the keyword ARRAY followed by the name of the array:
ARRAY array-name{ };
The array name is followed by either a pair of parentheses ( ), braces { }, or square brackets [ ]. In this article we use square braces { }.
/* syntax */
ARRAY ARRAY-NAME{SUBSCRIPT} ($) VARIABLE-LIST ARRAY-VALUES ;
Description:
- ARRAY is the SAS keyword to declare an array.
- ARRAY-NAME is the name of the array which follows the same rule as variable names.
- SUBSCRIPT is the number of values the array is going to store.
- ($) is an optional parameter to be used only if the array is going to store character values.
- VARIABLE-LIST is the optional list of variables which are the place holders for array values.
- ARRAY-VALUES are the actual values that are stored in the array. They can be declared here or can be read from a file or dataline.
Examples Of SAS Array Declaration
SAS Arrays can be declared in multiple ways depending on the data type and its values. But before that let’s discuss a few important things.
{*} : It specifies that SAS is to determine the length by counting the variables in the array
Variables
- _NUMERIC_ : It specifies all numeric variables.
- _CHARACTER_ : It specifies all character variables.
- _ALL_ : It specifies all variables.
_TEMPORARY_ : It creates a list of temporary data elements.
Here are some examples of SAS array declarations.
/* Declare an array of length 3 named “simple” with variables
named red, green, and yellow */
array simple{3} red green yellow;
/* Declare an array of length 7 named “days” with variables
named d1, d2, d3.., d7 */
array days{7} d1-d7;
/* Declare an array named “month” with variables named
jan,feb, jul, oct, nov. SAS will calculate length of an array by counting variables */
array month{*} jan feb jul oct nov;
/* Declare an array of length 5 named “rollno” with values 10, 20, 30, 40, 50 */
array rollno[5] (10 20 30 40 50);
/* Declare an array of length 4 named “test” with variables named
t1, t2, t3, t4 and its initial values 90,80,70,60 respectively */
array test{4} t1-t4 (90 80 70 60);
/* Declare an array of length 5 named “quests” which contain character values */
array quests(1:5) $ Q1-Q5;
/* Define an array and assign initial character values to the elements in the array */
array test2{*} $ a1 a2 a3 ('a','b','c');
Define Array With Numeric Variables
/* define array with var names */
array nvars nvars1-nvars5;
/* define array without var names. by default array name becomes var names */
array nvars {5} 8.;
The following example demonstrates how to create an array “num_array” with numeric variables named: num_var1, num_var2, num_var3, num_var4, num_var5 and the values are assigned in multiplications of 10.
/* create an array with numeric variables */
data num_array;
array nvars num_var1-num_var5;
do i=1 to 5;
nvars{i}=i*10;
end;
drop i;
run;
proc print data=num_array;
title 'method 1: create an array with numeric variables';
run;
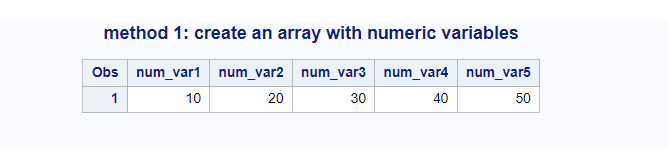
In the below example variable names are not specified in the array definition. It means by default array name (nvars) will be referred to as variable names as nvars1, nvars2, nvars3, nvars4, nvars5.
/* create an array with numeric variables */
data num_array;
array nvars {5} 8.;
do i=1 to dim(nvars);
nvars{i}=i * 10;
end;
drop i;
run;
proc print data=num_array;
title 'method 2: create an array with numeric variables';
run;
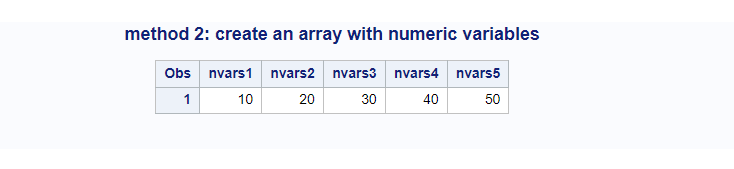
Define Array With Character Variables
/****** Define array with character variables ****/
/* define array with var names */
array cvars cvars1-cvars5;
/* define array without var names. by default array name becomes var names */
array cvars {5} $12.;
The following example demonstrates how to create and use an array (“cvars”) with character variables.
Here also there is no char variable names are mentioned it means array name will be referenced while creating character variable names as cvars1, cvars2, cvars3, cvars4, cvars5 with values “data_1”, “data_2”, “data_3”, “data_4”, “data_5” respectively.
/* create an array with character variables */
data char_array;
array cvars {5} $12.;
do i=1 to dim(cvars);
cvars{i}=cat('data_', i);
end;
drop i;
run;
proc print data=char_array;
title 'create an array with char variables';
run;
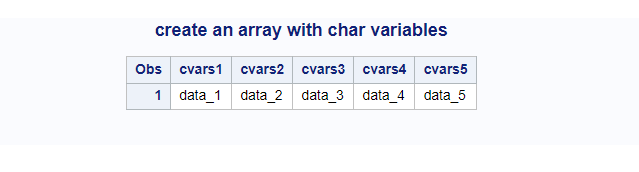
Programming Example 1: Compare and Set Values Using Arrays In SAS
In this example we are using the following sample dataset work.class. We will have a condition on age to compare the data. If the age is greater than 13 then update the AGE variable with blank values.
It is similar to using proc sql with UPDATE statement but here we are demonstrating this example with SAS arrays.
The array “nvars” is defined with the numeric variable “Age”. DIM() function is used to calculate length of the array.
/* create sample dataset */
data class(keep=Name Sex Age Height Weight);
set sashelp.class;
run;
proc print data=class;
title 'Dataset : work.class';
run;
/* Check If age greater than 13 then set blank Age */
data class_array;
set class;
array nvars (*) Age;
do i = 1 to dim(nvars);
if nvars{i} > 13 then nvars{i} =. ;
end;
drop i;
run;
/* view dataset */
proc print data=class_array;
title 'Dataset : work.class_array';
run;
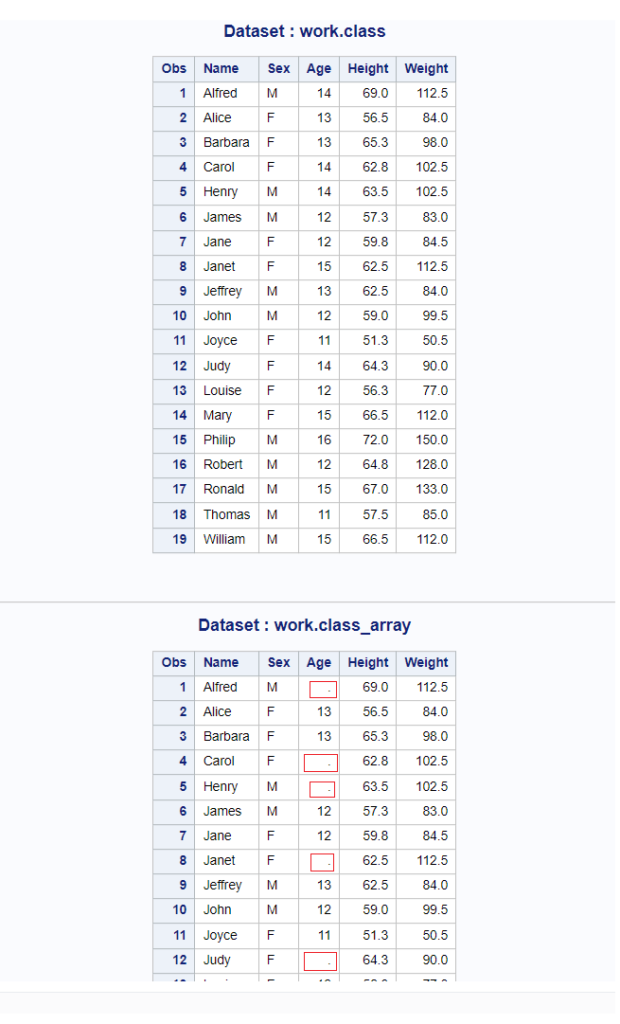
Programming Example 2: Multiply SAS Arrays
The sample dataset has three numeric variables: Age, Height, Weight. In the below example we have defined the following three SAS arrays.
- “nvars” array: It is defined for all three numeric variables Age, Height, and Weight
- “multiply” array: It’s a temporary array with values 1.1 , 1.2 ,1.3
- “calvars” array: It is defined for three numeric variables cal_age, cal_height, and cal_weight
The values for “calvars” array are assigned by multiplying “nvars” array with “multiply” array.
Multiply age by 1.1, Height by 1.2, and Weight by 1.3
/* multiply sas arrays */
data class_array;
set class;
array nvars (*) _numeric_;
array multiply {3} _temporary_ (1.1 , 1.2 ,1.3);
array calvars (*) cal_age cal_height cal_weight;
do i = 1 to dim(nvars);
/* multiple age by 1.1, Height by 1.2, and Weight by 1.3 */
calvars{i} = nvars{i} * multiply{i};
end;
drop i;
run;
/* view dataset */
proc print data=class_array;
run;
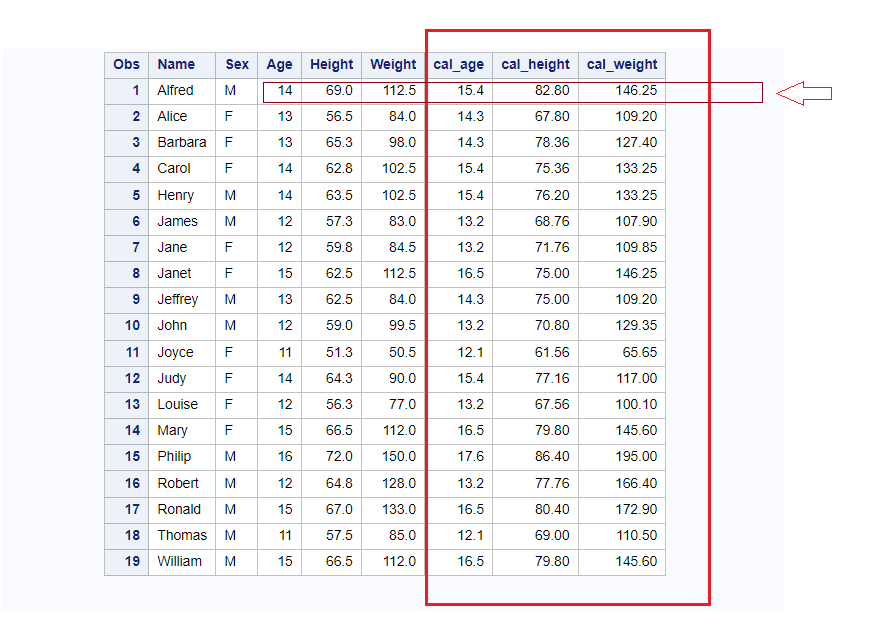
Programming Example 3: Calculate Statistics Of SAS Array Variables
You can calculate statistics of sum, mean, min, and max on array variables. You need to use the OF operator along with the statistics keyword. The calculation happens across the rows.
In the below example we have restricted the observation using obs=5 to demonstrate how statistics can be calculated using arrays in SAS.
/* Calculate statistics of SAS array variable */
data class_array;
set class (obs=5);
array nvars (*) _numeric_;
/* Calculate (sum of, mean of, max of, and min of) */
numVar_SUM = sum(of nvars(*));
numVar_MEAN = mean(of nvars(*));
numVar_MAX = max(of nvars(*));
numVar_MIN = min(of nvars(*));
run;
/* view dataset */
proc print data=class_array;
run;
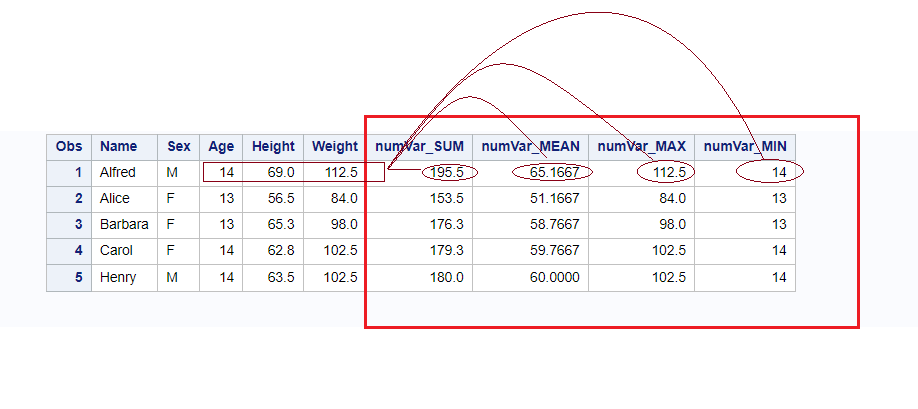
FAQ – How To Use Arrays In SAS
An array in SAS is a set of variables of the same type that you want to perform the same operation on.
Yes, all variables associated with an array must be of the same type, either character or numeric.
Arrays in SAS are used to simplify code, making programs more efficient and less error-prone.
They provide an alternative method for referring to a variable rather than using the name of the variable