We have already seen the multiple examples of SAS arrays declaration and in depth article on SAS Do loop. In this article we will demonstrate how to use the Do loop in SAS array.
Do Loop In SAS
In this method SAS executes statements between the DO and END statements repetitively, based on the value of an index variable.
Syntax:
DO index-variable=specification-1 <, ...specification-n>;
...more SAS statements...
END;
Here are some examples of how an iterative do loop works with the index variable.
/* iterative DO statements use a list of items for the value of start */
do month='JAN','FEB','MAR', ‘APR’, ‘MAY’;
do count=2,3,5,7,11,13,15,17;
do i='01JAN2050'd,'20FEB2050'd,'10APR2050'd;
/* iterative DO statements use the start TO stop */
do i=1 to 10;
do i=1 to y-5;
/* iterative DO statements use the BY increment */
do i=1 to 10 by 2;
do seq=2 to 8 by 2;
/* iterative DO statements use WHILE and UNTIL clauses */
do i=1 to 10 while(x<y);
do i=10 to 0 by -1 while(month='JAN');
How To Define SAS Arrays
A SAS array is a set of variables of the same type that you want to perform the same operation on. The variables in an array are called elements and can be accessed based on their position (also known as index).
You use the name of the array to reference the set of variables. Arrays are useful to create new variables and carry out repetitive tasks
Here are some examples of defining SAS arrays.
# Declare an array of length 3 named “simple” with variables named red, green, and yellow
array simple{3} red green yellow;
# Declare an array of length 7 named “days” with variables named d1, d2, d3.., d7
array days{7} d1-d7;
# Declare an array of length 5 named “rollno” with values 10, 20, 30, 40, 50.
array rollno[5] (10 20 30 40 50);
# Define an array and assign initial character values to the elements in the array.
array test2{*} $ a1 a2 a3 ('a','b','c');
Do Loop In SAS Array
In order to access each element of an array, and to update dataset or perform any operations can be done using the Do loop in SAS.
The following sample dataset will be used to demonstrate how to use do loop in SAS array.
/* create dataset */
data price_data;
set sashelp.pricedata;
run;
proc print data=price_data(obs=5);
run;

Example 1: How To Use Do Loop In SAS Array
If you observe in the above sample dataset, we have variables like price1, price2, price3, and so on..
In this example we will calculate a new price based on the existing prices multiplying by 1.2 using the Do loop in the SAS array.
- The SAS array “origprice” is defined for existing variables such as price1, price2, price3, etc.
- The SAS array “newprice” is defined for new variables to hold calculated new prices.
/* Example 1: do loop in sas array */
data pricedata_array;
set price_data;
array origprice price1-price17; /* define array for existing variables */
array newprice newprice1-newprice17; /* define array for new variables */
do over newprice;
newprice=origprice * 1.5;
end;
run;
/* view dataset */
proc print data=pricedata_array(obs=5);
var date sale price discount cost price1 newprice1 price2 newprice2;
run;
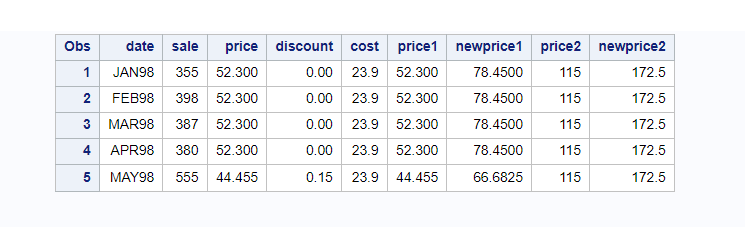
Example 2: How To Use Do Loop In SAS Array
The above code also can be written in different ways by using DIM(array-name) to tell SAS array length dynamically. Also, instead of writing “do over”, you can use index variable let’s say, “i” which can be written as “i=1 to dim(newprice)”
- The SAS array “origprice” is defined for existing numeric variables such as price1, price2, price3, etc.
- The SAS array “newprice” is defined for new variables to hold calculated new prices.
/* Example 2: do loop in sas array */
data pricedata_array;
set price_data;
array origprice {17} 8.; /* define array for existing variables */
array newprice {17} 8.; /* define array for new variables */
;
do i=1 to dim(newprice);
newprice{i}=origprice{i} * 1.5;
end;
run;
/* view dataset */
proc print data=pricedata_array(obs=5);
var date sale price discount cost price1 newprice1 price2 newprice2;
run;
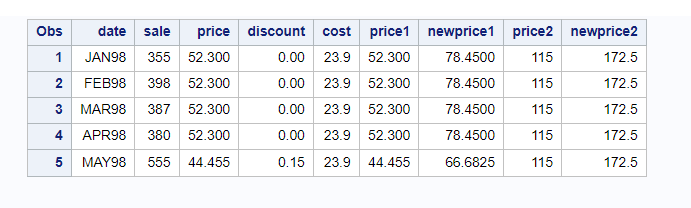