What Is SAS Loop?
A loop in SAS is a programming construct that is used to repeat a set of instructions for a specific number of times or until a certain condition is met.
There are three basic types of loops in SAS:
- DO Loop: This loop performs a certain number of iterations. For example, the loop do i = 1 to 5; y = i **2; output; end; will iterate 5 times, and the variable y will take on the values 1, 4, 9, 16, 25.
- DO WHILE Loop: This loop continues to iterate as long as a certain condition is true. The condition is checked before each iteration. For example, the loop do i = 1 to 10 while (x < 20); x = i*4; output; end; will stop iterating when the value of x reaches or exceeds 20.
- DO UNTIL Loop: This loop continues to iterate until a certain condition is met. The condition is checked after each iteration. For example, the loop do i = 1 to 10 until (x > 30); x = i*4; output; end; will stop iterating when the value of x exceeds 30.
These loops are fundamental to programming in SAS because they enable you to repeat a computation for various values of parameters.
1. DO Loop In SAS
The DO statement is the simplest form of DO group processing. The statements between the DO and END statements are called a DO group. You can nest DO statements within DO groups.
Syntax:
DO;
...more SAS statements...
END;
A simple DO statement is often used within IF-THEN/ELSE statements to designate a group of statements to be executed depending on whether the IF condition is true or false.
A. Iterative DO Loop
In this method SAS executes statements between the DO and END statements repetitively, based on the value of an index variable.
Syntax:
DO index-variable=specification-1 <, ...specification-n>;
...more SAS statements...
END;
Here are some examples of how an iterative do loop works with the index variable.
/* iterative DO statements use a list of items for the value of start */
do month='JAN','FEB','MAR', ‘APR’;
do count=2,3,5,7,11,13,15,17;
do i='01JAN2030'd,'25FEB2030'd,'18APR2030'd;
/* iterative DO statements use the start TO stop */
do i=1 to 10;
do i=1 to x-5;
/* iterative DO statements use the BY increment */
do i=1 to 10 by 2;
do count=2 to 8 by 2;
/* iterative DO statements use WHILE and UNTIL clauses */
do i=1 to 10 while(x<y);
do i=10 to 0 by -1 while(month='FEB');
Example 1: A Simple Iterative Do Loop In SAS
The following example shows how to create a SAS dataset using iterative do loop. The loop starts from 1 and ends at the 10th iteration and the index value is assigned to a variable “rank”.
/* create a new dataset with rank 1 to 10*/
data OnetoTen (keep=rank);
do i=1 to 10;
rank=i;
output;
end;
run;
/* view dataset */
proc print data=OnetoTen;
run;
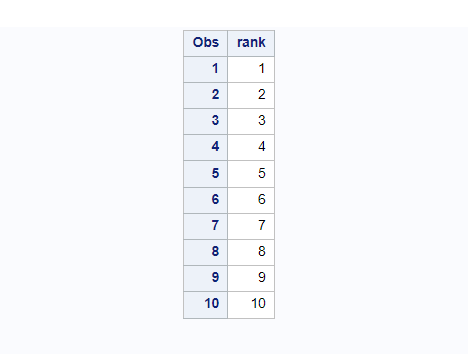
Example 2: How To Calculate Account Balance With Do Loop In SAS
In this example we are creating a new dataset “work.account” and maintaining the account balance. The initial balance is 4000 which is specified for the variable “Balance”.
The interactive loop starts with 1 and ends at 10th iteration. For every iteration we are adding +1000 into the total balance and writing the observation in the output dataset with an OUTPUT statement.
/* calculate account balance in sas */
data account;
Balance=4000;
do i=1 to 10;
balance=balance+1000;
output;
end;
run;
/* view dataset */
proc print data=account;
run;
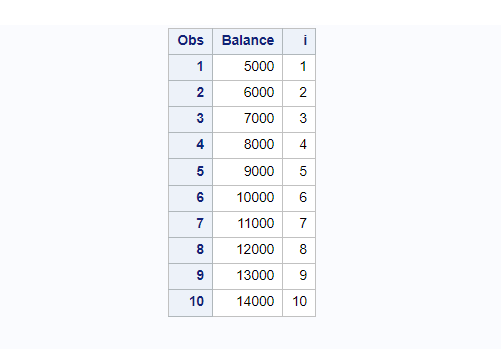
Example 3: Calculate Account Balance With Incremental Do Loop In SAS
The previous example was very straightforward to calculate account balance by adding 1000 into the balance. There is one thing that has changed in this example. We are using an incremental do loop here.
Instead of running a loop for 10 times starting at 1 and ending at 10, in this example the loop will run only 5 times due to the incremental option specified with the BY statement.
/* Calculate account balance with incremental do loop */
data account;
Balance=10000;
do i=1 to 10 by 2;
balance=balance+1000;
output;
end;
run;
/* view dataset */
proc print data=account;
run;
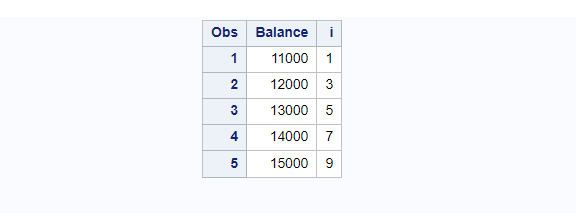
B. Nested Do Loop
Nested Do Loop means running an iterative do loop inside another iterative do loop.
It is commonly used when it comes to creating 2D or 3D tables. The most use cases can be found in the programs where SAS ARRAYS are being used.
In this example we have an inner loop with “j” as an index variable which starts at 1 and ends at 3. Whereas the outer loop has “i” as an index variable which starts at 1 and ends at 5.
For the demonstration we are assigning index variable values from both the inner and outer loop and writing the observations into the work.NestedDoLoop dataset.
/* nested do loop in sas */
data NestedDoLoop;
do i=1 to 5;
outerLoop=i;
do j=1 to 3;
innerLoop=j;
output;
end;
/* inner loop ends */
end;
/* outer loop ends */
drop i j;
run;
/* view dataset */
proc print data=NestedDoLoop;
run;
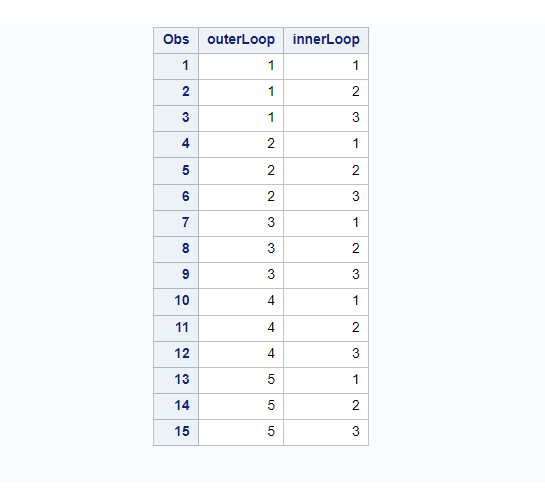
2. DO WHILE Loop In SAS
In the Do While Loop SAS executes statements in a DO loop repetitively while a condition is true.
Conditional Do While Loop statement:
- executes statements in the DO loop repetitively while a condition is true
- evaluates the condition at the top of the loop so that the DO WHILE loop never executes if the condition is false
Syntax:
DO <value> while(<condition>)...
END;
Example 1: A Simple Example of Do While Loop
In the below example “x” variable is initialized with the value 5. Do loop will execute until the condition (x < 10) mentioned in the WHILE statement is true. The OUTPUT statement is used to write the current observation to a SAS data set
/* do-while loop example */
data do_while;
x=5;
do while(x < 10);
x=x+1; output;
end;
run;
/* view dataset */
proc print data=do_while;
run;
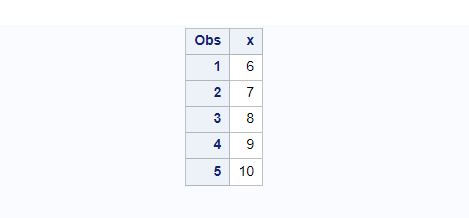
Example 2: Generate Loan Account Statement With Do While Loop
Here is how the sample loan account looks like: current loan amount is 10000, monthly EMI is 2000 and we are starting fresh from the first month of the EMI payment.
The below code calculates how many monthly EMI installments you need to pay to repay your entire loan amount. For the simplicity we have not considered principle and interest separately.
/* Generate loan account statement with Do-While Loop in sas*/
data loan_statement;
loan=10000;
EMI=2000;
payments=0;
do i=1 to 10 while(loan>0);
loan=loan-EMI;
payments=payments + 1;
output;
end;
run;
/* view dataset */
proc print data=loan_statement;
run;
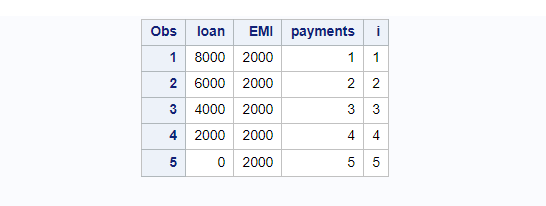
3. DO UNTIL Loop In SAS
In the Do Until Loop SAS executes statements in a DO loop repetitively until a condition is true.
Conditional Do Until Loop statement:
- executes statements in the DO loop repetitively until a condition is true
- evaluates the condition at the bottom of the loop so that the DO UNTIL loop always executes at least once, even if the condition is false.
Syntax:
Do until(<condition>)...
end;
Example 1: A Simple Example of Do Until Loop
In the below example “x” variable is initialized with the value 5. Do loop will execute until the condition (x < 10) mentioned in the UNTIL statement is true. The OUTPUT statement is used to write the current observation to a SAS data set.
/* do-until loop example */
data do_until;
x=5;
do until(x > 10);
x=x+1; output;
end;
run;
/* view dataset */
proc print data=do_until;
run;
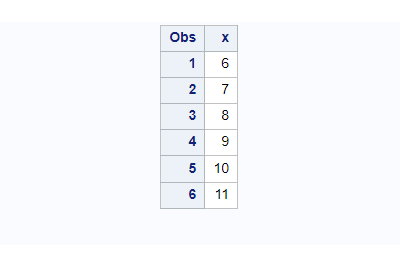
Example 2: Generate Loan Account Statement With Do Until Loop
Here is how the sample loan account looks like: current loan amount is 10000, monthly EMI is 2000 and we are starting fresh from the first month of the EMI payment.
We have already generated the loan statement using the Do-While loop. This example shows how to use do-until loop in SAS to generate the same statement.
You just need to use UNTIL statement instead of WHILE and mention following condition: until(loan=0)
/* Generate loan account statement with Do-Until Loop in SAS */
data loan_statement;
loan=10000;
EMI=2000;
payments=0;
do i=1 to 10 until(loan=0);
loan=loan-EMI;
payments=payments + 1;
output;
end;
run;
/* view dataset */
proc print data=loan_statement;
run;
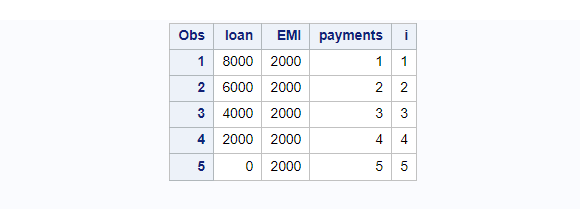
Example 3: Incremental Investment Until You Cross $500,000
This is one more yet realistic example of using an iterative do-loop in SAS to get the incremental investment details depending on your investment budget and the final target.
In this example your target is to hit a $500,000 investment fund. Let’s assume that you have $1000 initial amount that you have invested and year by year you’ll increase your investment by 2x. It means every year you’ll double down your investment amount.
First year you’ve invested $1,000 then the second year you’ll invest $2,000 and the next year $4,000 and so on..
/* incremental investment fund*/
data investment_fund;
fund=0;
investment=1000;
format fund dollar10.2;
do j=1 to 10 until(fund >=50000);
fund=fund + investment;
years=j;
output;
/* increase investment amount by 2x every year */
investment=investment*2;
end;
drop j;
run;
/* view dataset */
proc print data=investment_fund;
run;
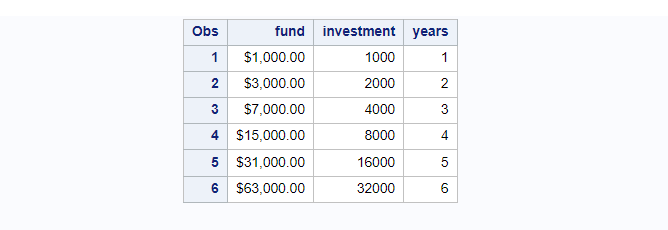
Comparison Between Do Loop, Do While, Do Until In SAS
- The DO statement, the simplest form of DO-group processing, designates a group of statements to be executed as a unit, usually as a part of IF-THEN/ELSE statements.
- The iterative DO statement executes statements between DO and END statements repetitively based on the value of an index variable.
- The DO UNTIL statement executes statements in a DO loop repetitively until a condition is true, checking the condition after each iteration of the DO loop. The DO WHILE statement evaluates the condition at the top of the loop; the DO UNTIL statement evaluates the condition at the bottom of the loop.
Note: If the expression is false, the statements in a DO WHILE loop do not execute. Because the DO UNTIL expression is evaluated at the bottom of the loop, the statements in the DO UNTIL loop always execute at least once.
FAQ – How To Use SAS Do Loop, Do While, and Do Until
A DO loop in SAS is a programming construct that repeats a set of instructions for a specific number of times
A DO WHILE loop in SAS continues to iterate as long as a certain condition is true
A DO UNTIL loop in SAS continues to iterate until a certain condition is met.
A DO loop performs a certain number of iterations, while a DO WHILE loop continues to iterate as long as a certain condition is true.