The PROC PRINT procedure is usually used to view dataset observations in SAS. You need to specify the dataset name with data=option in the proc print procedure.
Here is a simple example of how to print a dataset in SAS.
Basic Syntax:
proc print data=data-set-name;
run;
Here is the sample example to print sashelp.class dataset.
/* print sas dataset */
proc print data=sashelp.class;
title 'SAS Data set: sashelp.class';
run;
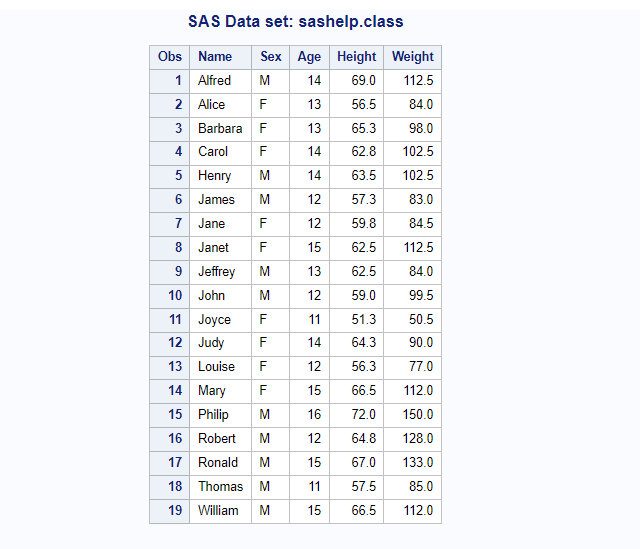
How To List Dataset Names From SAS Library
This is the first step to list dataset names to print all the datasets from a SAS library.
One way of doing this is to list dataset names manually. Another way is to use dictionary.tables in the proc sql procedure.
Dictionary.TABLES
When you need more information about SAS files consider using the TABLES dictionary table.
The TABLES dictionary table provides detailed information about the library name, member name and type, date created and last modified, number of observations, observation length, number of variables, password protection, compression, encryption, number of pages, reuse space, buffer size, number of deleted observations, type of indexes, and requirements vector.
For example, to obtain a detailed list of dataset in the SASHELP library, the following code can be used.
/* create table with list of datasets from sas lib */
proc sql;
create table table_list as select * from dictionary.tables
where libname=upcase("SASHELP") and nobs ne 0;
quit;
/* view dataset */
proc print data=table_list; run;
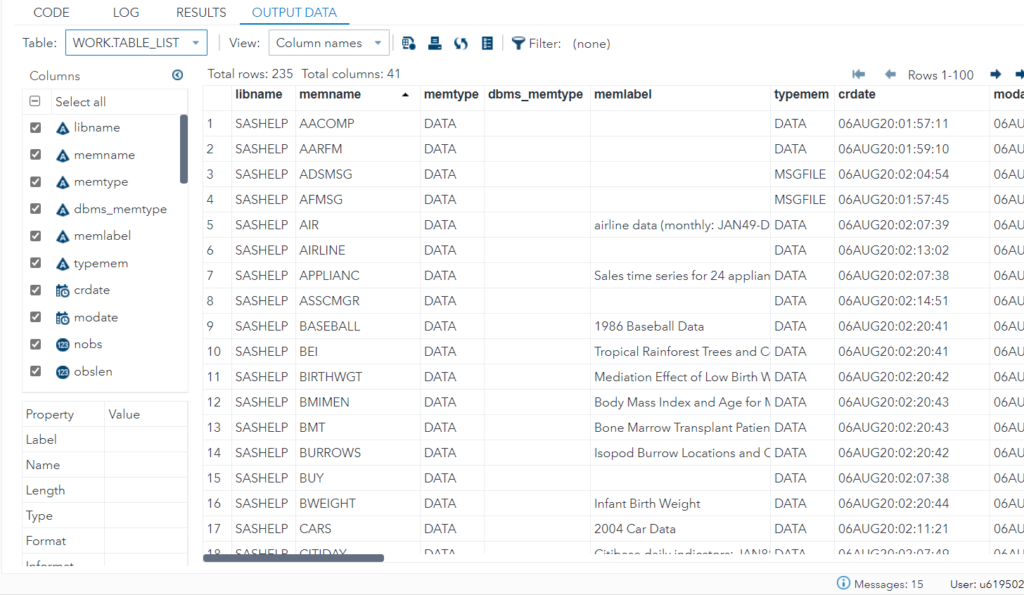
How To Print Datasets
Now you can use the work.table_list dataset to print datasets using table names from memname column.
The best way to print datasets is to use the CALL EXECUTE routine with data _null_ statement using the dataset work.table_list values by passing the argument.
This example prints only three sample datasets, five observations from each. You can remove the obs=5 statement to print entire datasets.
/* print sample three datasets */
data _null_;
set table_list (obs=3);
call execute(cats(
'proc print data=sashelp.', memname, '(obs=5) ;',
'title Data set: sashelp.', memname,
'; run;'));
run;
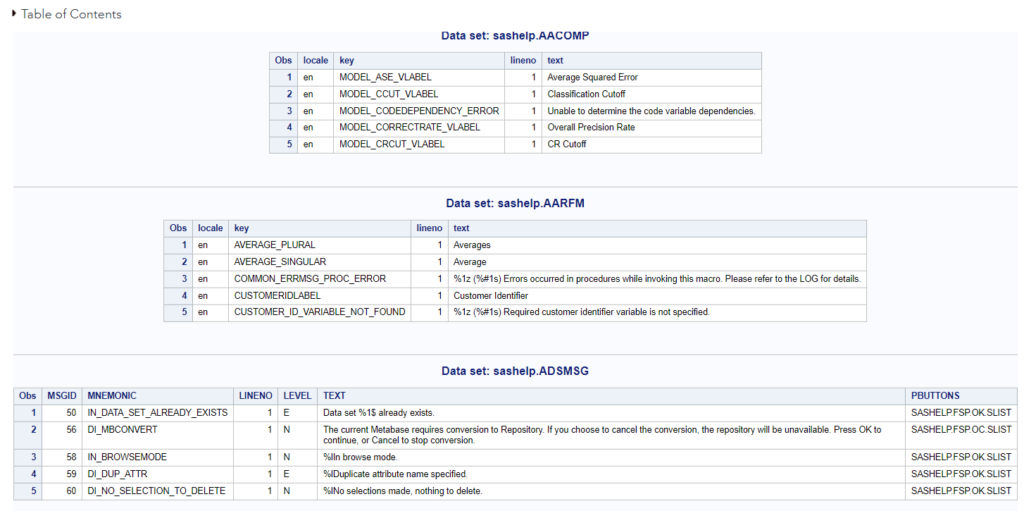
How To Print All The Datasets From A SAS Library
Let’s standardize the above code by creating a SAS macro that will print all the datasets from a SAS library dynamically. If you look at the first example where we have hard-coded library name “SASHELP” to create table_list dataset.
The below SAS macro takes the two input parameters, printlib and worklib. The printlib is the library that you want to list the dataset names and print all the datasets using call execute routine.
In this example we have used again SASHELP library reference for the demonstration but if you have a base library then it can be defined in the beginning. You can refer to the commented library statement.
/*********************************************
Print all the datasets from a SAS library
***************************************************/
/* libname printlib 'SAS-data-library'; */
%let printlib=%upcase(sashelp); /* set print library */
%put &printlib;
options nodate pageno=1;
%macro printall(libname, worklib);
proc sql;
create table &worklib..table_list as select * from dictionary.tables where
libname=upcase("&libname.") and nobs ne 0;
quit;
data _null_;
set &worklib..table_list(obs=3);
call execute(cats(
'proc print data=&libname..', memname, '(obs=5);',
' title Data set: &libname..', memname,
'; run;'));
run;
%mend printall;
%printall(libname= &printlib, worklib=work);
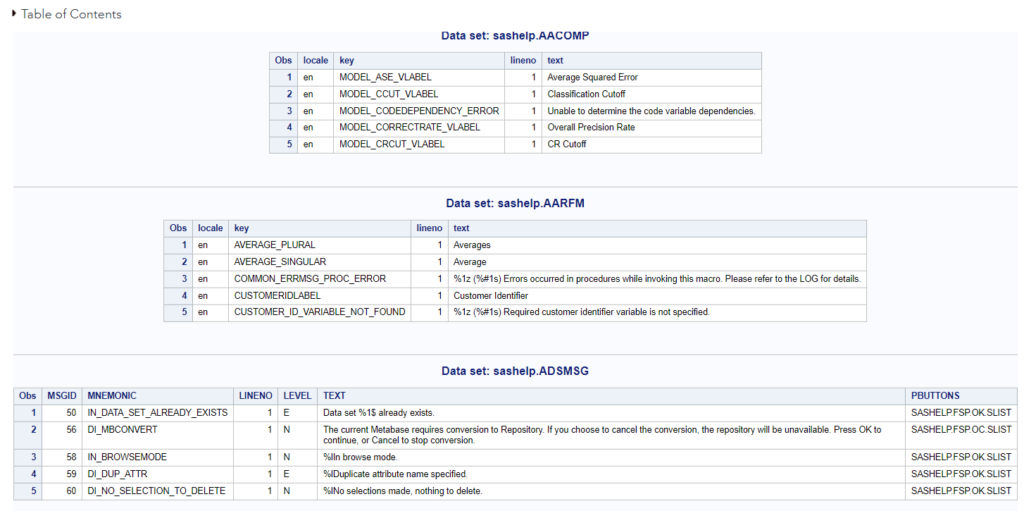
FAQ – How To Print All The Datasets In SAS
You can use PROC PRINT with the following syntax to print every row in the dataset:
proc print data=my_data; run;
You can use the same programming logic with any procedure. Just replace the PROC PRINT step near the end of the example with whatever procedure step you want to execute.
Alternatively, you can use dictionary.tables to list table names and pass it into proc print procedure through CALL Execute routine. It will print all the datasets from that list.